In the ever-evolving landscape of data analysis, mastering Python has become a key asset for professionals and enthusiasts alike. Its versatility and powerful libraries make Python a top choice for handling data with efficiency and precision. Today, we dive deep into the realm of mastering data analysis in Python, equipping you with the tools and knowledge to unlock its full potential.
Getting Started with Python for Data Analysis
To embark on your journey to mastering data analysis with Python, understanding the basics is crucial. Python's intuitive syntax and readability make it an ideal starting point, even for beginners. With libraries like Pandas, NumPy, and Matplotlib at your disposal, you have a comprehensive toolkit for performing data manipulation, statistical analysis, and visualization.
Exploring Data with Pandas
At the core of Python's data analysis capabilities lies Pandas, a powerful library for working with structured data. Whether you're importing data from CSV files or connecting to databases, Pandas offers versatile tools for cleaning, transforming, and aggregating datasets.
Example: Loading and Exploring Data with Pandas**
```python
import pandas as pd
# Load a CSV file into a DataFrame
df = pd.read_csv('data.csv')
# Display the first few rows
print(df.head())
# Basic statistics
print(df.describe())
```
This code snippet demonstrates how to load a dataset into a Pandas DataFrame, view the first few rows, and get a summary of basic statistics.
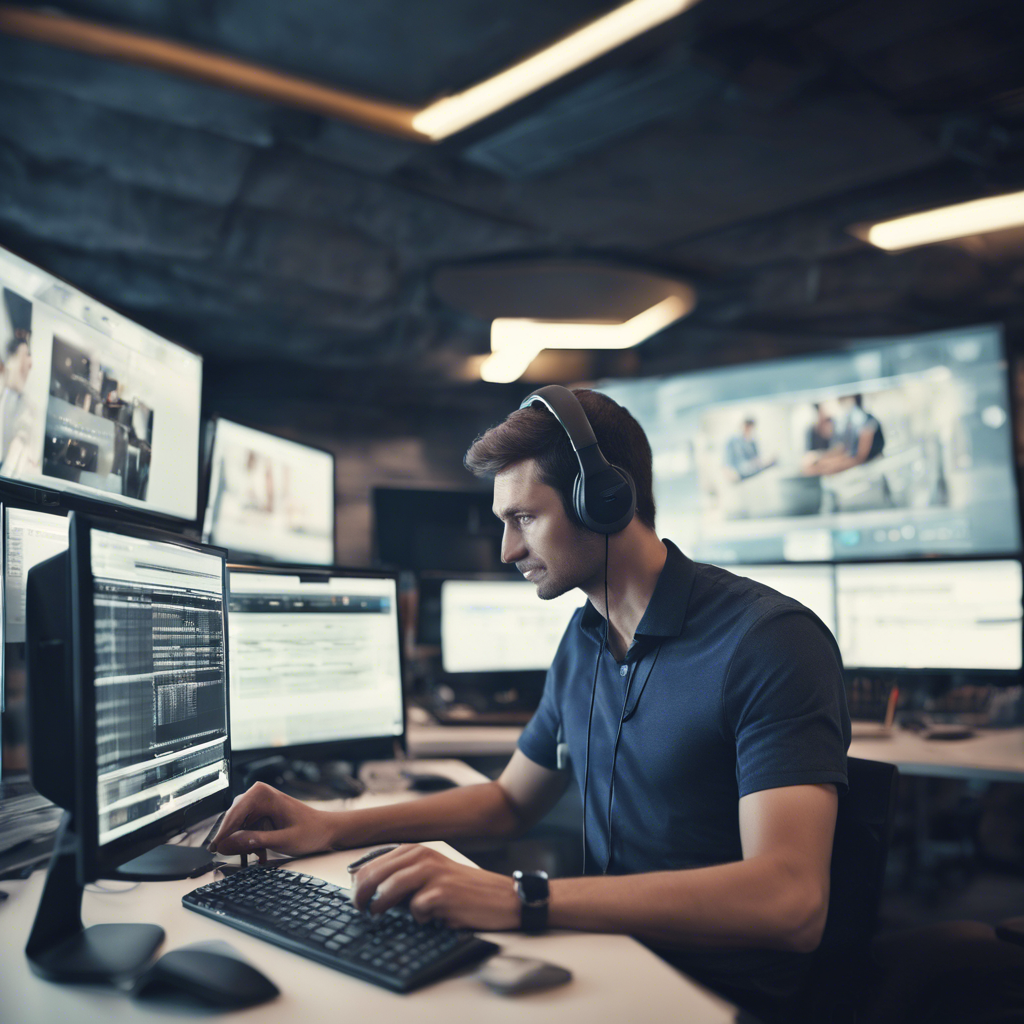
Analyzing Data with NumPy
When it comes to numerical computing, NumPy is a go-to library in Python. With its support for multi-dimensional arrays and mathematical functions, NumPy simplifies complex operations and enhances performance. From handling matrices to vectorized calculations, NumPy streamlines data analysis tasks efficiently.
Example: Numerical Operations with NumPy**
```python
import numpy as np
# Create a NumPy array
data = np.array([1, 2, 3, 4, 5])
# Perform basic operations
print("Mean:", np.mean(data))
print("Standard Deviation:", np.std(data))
print("Sum:", np.sum(data))
```
This snippet shows how to create a NumPy array and perform basic statistical operations on the data.
Visualizing Insights with Matplotlib
Visualizing data is essential for interpreting trends and patterns effectively. Matplotlib, a versatile plotting library in Python, empowers you to create insightful visualizations, ranging from bar charts and histograms to scatter plots and heatmaps. By customizing colors, labels, and styles, you can craft compelling visual representations of your data.
Example: Creating a Simple Bar Chart with Matplotlib**
```python
import matplotlib.pyplot as plt
# Sample data
categories = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
# Create a bar chart
plt.bar(categories, values)
plt.xlabel('Category')
plt.ylabel('Value')
plt.title('Sample Bar Chart')
plt.show()
```
This snippet illustrates how to create a basic bar chart using Matplotlib.
Going Beyond with Advanced Techniques
As you progress in your Python data analysis journey, exploring advanced techniques can elevate your skills to new heights. Machine learning algorithms, such as regression and clustering, offer predictive capabilities, while deep learning models enable complex pattern recognition. By delving into these advanced concepts, you can unlock the full potential of Python for data analysis.
Example: Simple Linear Regression with scikit-learn**
```python
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1, 3, 2, 5, 4])
# Create and fit the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(X)
print("Predictions:", predictions)
```
This snippet demonstrates how to perform a simple linear regression using the scikit-learn library.
Optimizing Performance with Python
To maximize efficiency in large-scale data analysis projects, optimizing the performance of your Python code is essential. Techniques like parallel processing, using optimized libraries, and minimizing memory usage can significantly enhance the speed and scalability of your data analysis workflows.
Example: Parallel Processing with Joblib**
```python
from joblib import Parallel, delayed
import numpy as np
# Function to be parallelized
def process_data(x):
return x * x
# Sample data
data = np.arange(10)
# Parallel processing
results = Parallel(n_jobs=4)(delayed(process_data)(i) for i in data)
print("Results:", results)
```
This snippet shows how to use the Joblib library to parallelize a simple function for faster processing.
Embracing the Future of Data Analysis
With Python shaping the future of data analysis, staying abreast of emerging trends and technologies is paramount. From cloud computing and big data processing to artificial intelligence and automation, Python continues to evolve, offering endless possibilities for data enthusiasts.
Conclusion
In conclusion, mastering data analysis in Python opens doors to a world of opportunities, where insights are waiting to be uncovered and decisions are waiting to be influenced. By harnessing the power of Python's libraries and tools, you can transform raw data into actionable insights, making informed decisions that drive success.
So, are you ready to embark on your Python data analysis journey? Let Python be your guiding light in the vast landscape of data analytics, leading you towards a brighter, more data-driven future.
Remember, the power is in your hands. Unleash it with Python.
Comments